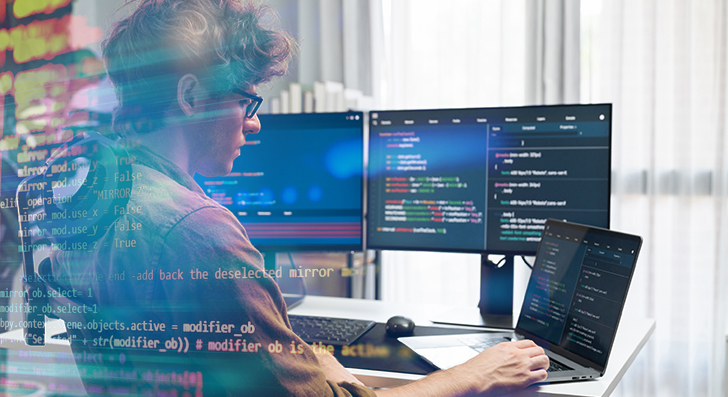
Scalability implies your software can take care of progress—much more users, additional knowledge, and even more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part within your program from the start. Several purposes fall short every time they increase fast due to the fact the original layout can’t handle the extra load. For a developer, you might want to Believe early regarding how your program will behave stressed.
Begin by coming up with your architecture to become versatile. Steer clear of monolithic codebases wherever every thing is tightly related. Rather, use modular style or microservices. These styles break your app into smaller, unbiased parts. Just about every module or services can scale By itself without the need of influencing the whole technique.
Also, give thought to your database from day just one. Will it need to deal with 1,000,000 people or maybe 100? Pick the correct variety—relational or NoSQL—dependant on how your data will expand. Prepare for sharding, indexing, and backups early, Even when you don’t need to have them yet.
An additional vital point is to avoid hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Think of what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like message queues or occasion-driven systems. These help your application tackle extra requests without the need of having overloaded.
After you Establish with scalability in mind, you are not just planning for achievement—you happen to be lowering potential headaches. A well-prepared process is less complicated to keep up, adapt, and expand. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a essential Portion of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as bring about failures as your app grows.
Get started by knowledge your info. Can it be remarkably structured, like rows in a very table? If Sure, a relational databases like PostgreSQL or MySQL is a great match. These are definitely sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like read replicas, indexing, and partitioning to take care of a lot more traffic and information.
In the event your info is a lot more flexible—like consumer exercise logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at handling substantial volumes of unstructured or semi-structured data and might scale horizontally extra very easily.
Also, consider your go through and generate patterns. Do you think you're doing many reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that will cope with high compose throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not want Innovative scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Keep away from pointless joins. Normalize or denormalize your info dependant upon your access patterns. And usually keep an eye on databases functionality while you increase.
Briefly, the appropriate databases relies on your application’s structure, speed needs, and how you expect it to grow. Take time to select sensibly—it’ll help save many issues later on.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each little hold off provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your system. That’s why it’s crucial to build economical logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most sophisticated Resolution if a simple a person will work. Maintain your capabilities limited, targeted, and straightforward to test. Use profiling tools to uncover bottlenecks—spots exactly where your code requires much too prolonged to run or works by using excessive memory.
Following, take a look at your databases queries. These frequently gradual items down more than the code by itself. Ensure that Each and every question only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of performing a lot of joins, especially across substantial tables.
In the event you observe the same knowledge remaining requested over and over, use caching. Retail store the outcomes quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app extra effective.
Remember to examination with substantial datasets. Code and queries that work good with 100 information may possibly crash every time they have to take care of one million.
To put it briefly, scalable apps are rapidly applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more buyers and more visitors. If every thing goes via a single server, it's going to swiftly become a bottleneck. That’s in which load balancing and caching can be found in. Both of these equipment aid maintain your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the do the job, the load balancer routes people to diverse servers depending on availability. This implies no one server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar information yet again—like a product web site or possibly a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces databases load, enhances speed, and can make your app much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app manage a lot more people, stay quickly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you may need instruments that permit your app develop conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you'll need them. You don’t need to acquire hardware or guess foreseeable future potential. When visitors raises, you'll be able to incorporate far more assets with just a couple clicks or routinely working with automobile-scaling. When targeted traffic drops, it is possible to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You can center on making your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one device. This makes it easy to maneuver your application among environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when issues transpire. If you would like your application to expand without limitations, get started making use of these instruments early. They save time, lessen risk, and allow you to continue to be centered on developing, not fixing.
Check All the things
Should you don’t observe your application, you gained’t know when points go wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of building scalable methods.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it will require for people to load internet pages, how frequently faults materialize, and where they occur. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Setup alerts for essential issues. For instance, If the reaction time goes earlier mentioned a limit or even a support website goes down, you ought to get notified right away. This aids you resolve problems quick, often before buyers even detect.
Checking is additionally helpful when you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again ahead of it triggers genuine destruction.
As your application grows, site visitors and data raise. With no monitoring, you’ll miss out on signs of trouble until eventually it’s also late. But with the proper applications in position, you continue to be in control.
To put it briefly, monitoring can help you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your program and making sure it works well, even under pressure.
Remaining Ideas
Scalability isn’t only for huge companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and using the appropriate tools, it is possible to Establish apps that improve smoothly with no breaking stressed. Begin smaller, think massive, and Develop sensible.